File System Interface
Page Contents
- File Concept
- Access Methods
- Directory Structure
- File System Mounting
- File Sharing
- Protection
File Concept
- Contiguous logical address space
Types:
- Data
- numeric
- character
- binary
- Program
File Structure
- None – sequence of words, bytes
- Simple record structure
- Lines
- Fixed length
- Variable length
- Complex Structures
- Formatted document
- Relocatable load file
- Can simulate last two with first method by inserting appropriate control characters
- Who decides:
- Operating system
- Program
File Attributes
Name: only information kept in human-readable form.
Type: needed for systems that support different types.
Location: pointer to file location on device
Size: current file size
Protection: controls who can do reading, writing, execution.
Time, date, and user identification:data for protection, security, and usage monitoring
Information about files are kept in the directory structure, which is maintained on the disk
File Operations
Create
Write
Read
File seek – reposition within file
Delete
Truncate
Open(Fi) – search the directory structure on disk for entry Fi, and move the content of entry to memory
Close (Fi) – move the content of entry Fi in memory to directory structure on disk
Open Files:
Several pieces of data are needed to manage open files:
- File pointer: pointer to last read/write location, per process that has the file open
- File-open count: counter of number of times a file is open – to allow removal of data from open-file table when last processes closes it
- Disk location of the file: cache of data access information
- Access rights: per-process access mode information
Open File Locking:
- Provided by some operating systems and file systems
- Mediates access to a file
- Mandatory or advisory
- Mandatory – access is denied depending on locks held and requested
- Advisory – processes can find status of locks and decide what to do
File Locking Example – Java API
import java.io.*; import java.nio.channels.*; public class LockingExample { public static final boolean EXCLUSIVE = false; public static final boolean SHARED = true; public static void main(String arsg[]) throws IOException { FileLock sharedLock = null; FileLock exclusiveLock = null; try { RandomAccessFile raf = new RandomAccessFile("file.txt", "rw"); // get the channel for the file FileChannel ch = raf.getChannel(); // this locks the first half of the file - exclusive exclusiveLock = ch.lock(0, raf.length()/2, EXCLUSIVE); /** Now modify the data . . . */ // release the lock exclusiveLock.release(); // this locks the second half of the file - shared sharedLock = ch.lock(raf.length()/2+1, raf.length(), SHARED); /** Now read the data . . . */ // release the lock exclusiveLock.release(); } catch (java.io.IOException ioe) { System.err.println(ioe); }finally { if (exclusiveLock != null) exclusiveLock.release(); if (sharedLock != null) sharedLock.release(); } } } Copy the above code import java.io.*; import java.nio.channels.*; public class LockingExample { public static final boolean EXCLUSIVE = false; public static final boolean SHARED = true; public static void main(String arsg[]) throws IOException { FileLock sharedLock = null; FileLock exclusiveLock = null; try { RandomAccessFile raf = new RandomAccessFile("file.txt", "rw"); // get the channel for the file FileChannel ch = raf.getChannel(); // this locks the first half of the file - exclusive exclusiveLock = ch.lock(0, raf.length()/2, EXCLUSIVE); /** Now modify the data . . . */ // release the lock exclusiveLock.release(); // this locks the second half of the file - shared sharedLock = ch.lock(raf.length()/2+1, raf.length(), SHARED); /** Now read the data . . . */ // release the lock exclusiveLock.release(); } catch (java.io.IOException ioe) { System.err.println(ioe); }finally { if (exclusiveLock != null) exclusiveLock.release(); if (sharedLock != null) sharedLock.release(); } } }
File Types – Name, Extension
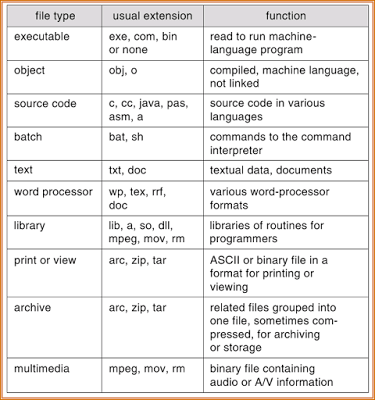
Access Methods:
Sequential Access:
read next
write next
reset
no read after last write
(rewrite)
Direct Access:
read n
write n
position to n
read next
write next
rewrite n
n = relative block number
Sequential-access File:
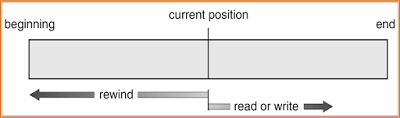
Simulation of Sequential Access on a Direct-access File
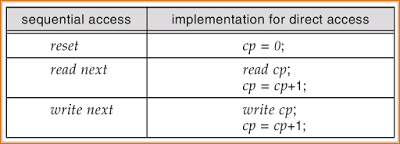
Example of Index and Relative Files
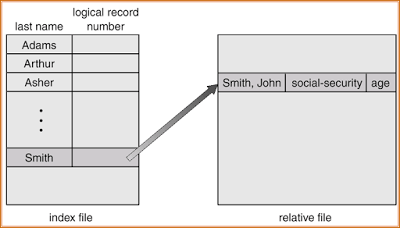
Directory Structure
A collection of nodes containing information about all files
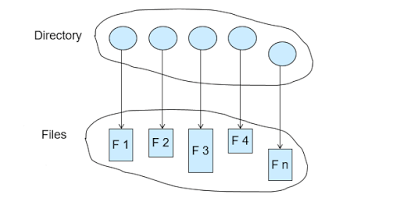
Both the directory structure and the files reside on disk
Backups of these two structures are kept on tapes
A Typical File-system Organization
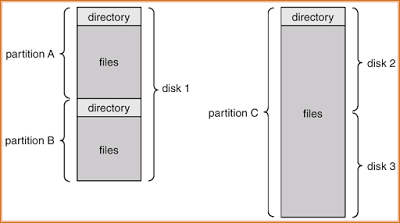
Information in a Device Directory
- Name
- Type
- Address
- Current length
- Maximum length
- Date last accessed (for archival)
- Date last updated (for dump)
- Owner ID
- Protection information (discuss later)
Operations Performed on Directory
- Search for a file
- Create a file
- Delete a file
- List a directory
- Rename a file
- Traverse the file system
Organize the Directory (Logically) to Obtain:
- Efficiency – locating a file quickly
- Naming – convenient to users
- Two users can have same name for different files
- The same file can have several different names
- Grouping – logical grouping of files by properties, (e.g., all Java programs, all games, …)
Single-Level Directory
A single directory for all users
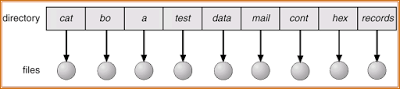
Naming
problem
problem
Grouping
problem
problem
Two-Level Directory
Separate directory for each user
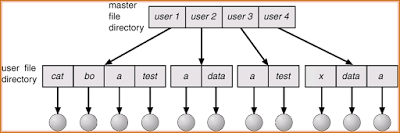
- Path name
- Can have the same file name for different user
- Efficient searching
- No grouping capability
Tree-Structured Directories
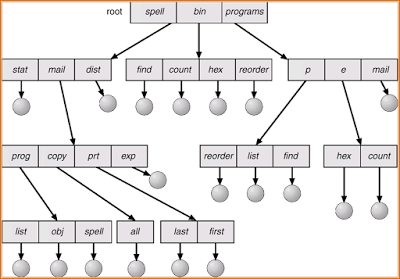
Efficient searching
Grouping Capability
Current directory (working directory)
- cd /spell/mail/prog
- type list
Absolute or relative path name
Creating a new file is done in current directory
Delete a file
rm <file-name>
Creating a new subdirectory is done in current directory
mkdir <dir-name>
Example: if in current directory /mail
mkdir count

Deleting “mail” deleting the entire subtree rooted by “mail”
Acyclic-Graph Directories:
Have shared subdirectories and files
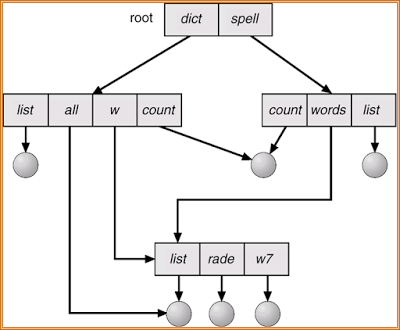
- Two different names (aliasing)
- If dict deletes list dangling pointer
Solutions:
- Backpointers, so we can delete all pointers Variable size records a problem
- Backpointers using a daisy chain organization
- Entry-hold-count solution
General Graph Directory
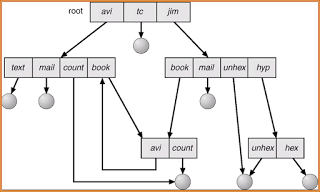
- How do we guarantee no cycles?
- Allow only links to file not subdirectories
- Garbage collection
- Every time a new link is added use a cycle detection algorithm to determine whether it is OK
File System Mounting
- A file system must be mounted before it can be accessed
- A unmounted file system is mounted at a mount point
(a) Existing. (b) Unmounted Partition
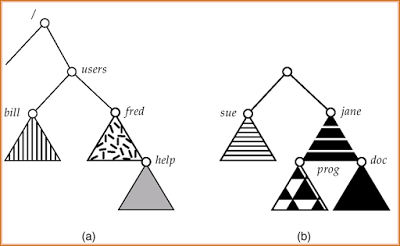
Mount Point
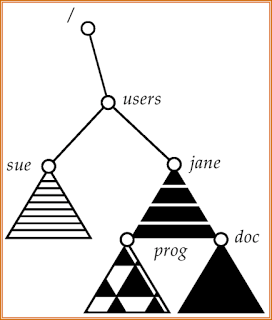
File Sharing
-
Sharing of files on multi-user systems is desirable
-
Sharing may be done through a protection scheme
-
On distributed systems, files may be shared across a network
-
Network File System (NFS) is a common distributed file-sharing method
File Sharing – Multiple Users
User IDs identify users, allowing permissions and protections to be per-user
Group IDs allow users to be in groups, permitting group access rights
File Sharing – Remote File Systems
-
Uses networking to allow file system access between systems
-
Manually via programs like FTP
-
Automatically, seamlessly using distributed file systems
-
Semi automatically via the world wide web
Client-server model allows clients to mount remote file systems from servers
- Server can serve multiple clients
- Client and user-on-client identification is insecure or complicated
- NFS is standard UNIX client-server file sharing protocol
- CIFS is standard Windows protocol
- Standard operating system file calls are translated into remote calls
Distributed Information Systems (distributed naming services) such as LDAP, DNS, NIS implement unified access to information needed for remote computing
File Sharing – Failure Modes
- Remote file systems add new failure modes, due to network failure, server failure
- Recovery from failure can involve state information about status of each remote request
- Stateless protocols such as NFS include all information in each request, allowing easy recovery but less security
File Sharing – Consistency Semantics
Consistency semantics specify how multiple users are to access a shared file simultaneously
- Similar to Ch 7 process synchronization algorithms
- Tend to be less complex due to disk I/O and network latency (for remote file systems
- Andrew File System (AFS) implemented complex remote file sharing semantics
- Unix file system (UFS) implements:
- Writes to an open file visible immediately to other users of the same open file
- Sharing file pointer to allow multiple users to read and write concurrently
- AFS has session semantics
- Writes only visible to sessions starting after the file is closed
Protection
File owner/creator should be able to control:
- what can be done
- by whom
Types of access Read
- Write
- Execute
- Append
- Delete
- List
Access Lists and Groups
Mode of access: read, write, execute
Three classes of users
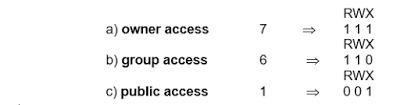
Ask manager to create a group (unique name), say G, and add some users to the group.
For a particular file (say game) or subdirectory, define an appropriate access.
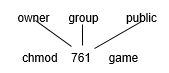
Your questions and feedback is valuable to us and we will be more than happy to answer your queries. Like our Facebook Page.
More related posts:
1) Linkers and loaders
2)Two pass assemblers